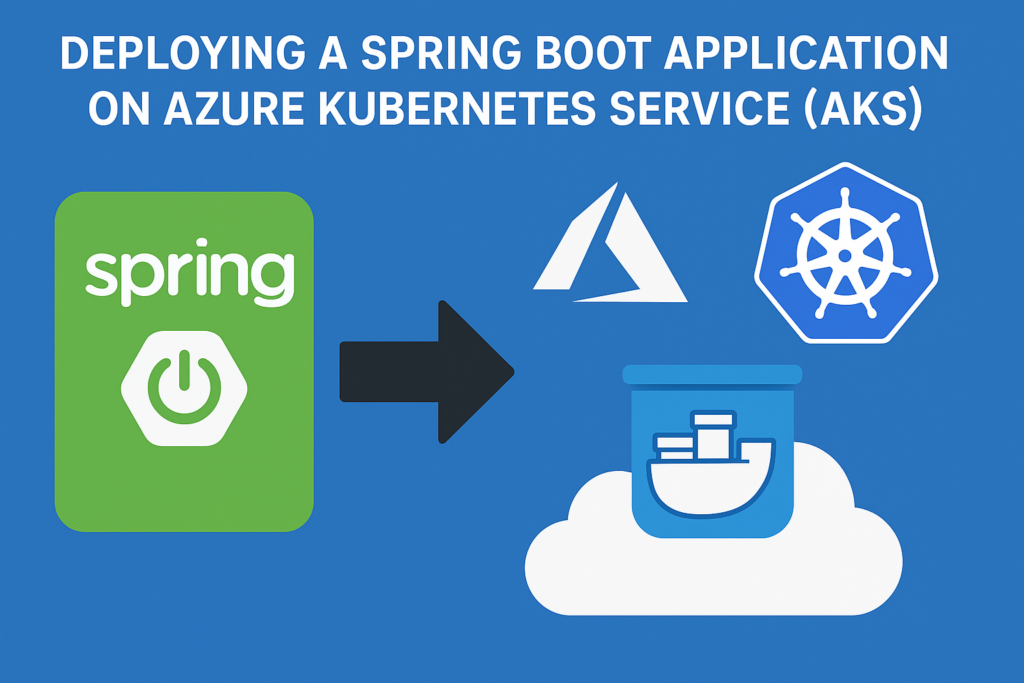
This step-by-step guide will walk you through deploying a Spring Boot application on Azure Kubernetes Service (AKS), covering everything from initial setup to production deployment.
Prerequisites
- Azure account with active subscription
- Azure CLI installed (installation guide)
- Docker installed
- kubectl installed
- Maven or Gradle (depending on your build tool)
- JDK 11 or later
Step 1: Create a Spring Boot Application
If you don't already have a Spring Boot application, create one:
# Using Spring Initializr (https://start.spring.io/)
# Or with curl:
curl https://start.spring.io/starter.zip -d dependencies=web,actuator -d javaVersion=11 -d type=maven-project -d bootVersion=2.7.0 -d groupId=com.example -d artifactId=aks-demo -o aks-demo.zip
unzip aks-demo.zip
cd aks-demo
Step 2: Containerize Your Spring Boot Application
Create a Dockerfile in your project root:
# Dockerfile
FROM eclipse-temurin:17-jdk-jammy as builder
WORKDIR /app
COPY .mvn/ .mvn
COPY mvnw pom.xml ./
RUN ./mvnw dependency:go-offline
COPY src ./src
RUN ./mvnw clean package -DskipTests
FROM eclipse-temurin:17-jre-jammy
WORKDIR /app
COPY --from=builder /app/target/*.jar app.jar
EXPOSE 8080
ENTRYPOINT ["java", "-jar", "app.jar"]
Build the Docker image:
docker build -t springboot-aks-demo .
Test the container locally:
docker run -p 8080:8080 springboot-aks-demo
Step 3: Push Image to Azure Container Registry (ACR)
3.1 Login to Azure CLI
az login
3.2 Create a Resource Group
az group create --name springboot-aks-rg --location eastus
3.3 Create Azure Container Registry
az acr create --resource-group springboot-aks-rg \
--name springbootaksacr \
--sku Basic \
--admin-enabled true
3.4 Login to ACR
az acr login --name springbootaksacr
3.5 Tag and Push Image to ACR
# Get ACR login server name
ACR_LOGIN_SERVER=$(az acr show --name springbootaksacr --query loginServer --output tsv)
# Tag the image
docker tag springboot-aks-demo $ACR_LOGIN_SERVER/springboot-aks-demo:v1
# Push the image
docker push $ACR_LOGIN_SERVER/springboot-aks-demo:v1
Step 4: Create Azure Kubernetes Service (AKS) Cluster
4.1 Create AKS Cluster
az aks create \
--resource-group springboot-aks-rg \
--name springboot-aks-cluster \
--node-count 2 \
--generate-ssh-keys \
--attach-acr springbootaksacr \
--node-vm-size Standard_B2s
4.2 Get AKS Credentials
az aks get-credentials --resource-group springboot-aks-rg --name springboot-aks-cluster
4.3 Verify Cluster Connection
kubectl get nodes
Step 5: Deploy Spring Boot Application to AKS
5.1 Create Kubernetes Deployment Manifest
Create deployment.yaml
:
# deployment.yaml
apiVersion: apps/v1
kind: Deployment
metadata:
name: springboot-app
spec:
replicas: 2
selector:
matchLabels:
app: springboot-app
template:
metadata:
labels:
app: springboot-app
spec:
containers:
- name: springboot-app
image: springbootaksacr.azurecr.io/springboot-aks-demo:v1
ports:
- containerPort: 8080
readinessProbe:
httpGet:
path: /actuator/health
port: 8080
initialDelaySeconds: 10
periodSeconds: 5
livenessProbe:
httpGet:
path: /actuator/health
port: 8080
initialDelaySeconds: 20
periodSeconds: 10
resources:
requests:
cpu: "500m"
memory: "512Mi"
limits:
cpu: "1000m"
memory: "1024Mi"
5.2 Create Kubernetes Service Manifest
Create service.yaml
:
# service.yaml
apiVersion: v1
kind: Service
metadata:
name: springboot-app-service
spec:
type: LoadBalancer
ports:
- port: 80
targetPort: 8080
selector:
app: springboot-app
5.3 Deploy to AKS
kubectl apply -f deployment.yaml
kubectl apply -f service.yaml
5.4 Verify Deployment
kubectl get pods
kubectl get deployments
kubectl get services
Step 6: Access Your Application
Get the external IP:
kubectl get service springboot-app-service --watch
Once the EXTERNAL-IP is assigned, access your application at http://<EXTERNAL-IP>
Step 7: Configure Ingress (Optional)
7.1 Install NGINX Ingress Controller
kubectl apply -f https://raw.githubusercontent.com/kubernetes/ingress-nginx/controller-v1.1.1/deploy/static/provider/cloud/deploy.yaml
7.2 Create Ingress Resource
Create ingress.yaml
:
# ingress.yaml
apiVersion: networking.k8s.io/v1
kind: Ingress
metadata:
name: springboot-app-ingress
annotations:
nginx.ingress.kubernetes.io/rewrite-target: /
spec:
rules:
- http:
paths:
- path: /
pathType: Prefix
backend:
service:
name: springboot-app-service
port:
number: 80
Apply the ingress:
kubectl apply -f ingress.yaml
Get the ingress IP:
kubectl get ingress
Step 8: Scaling Your Application
9.1 Manual Scaling
kubectl scale deployment springboot-app --replicas=3
9.2 Horizontal Pod Autoscaler
Create hpa.yaml
:
apiVersion: autoscaling/v2beta2
kind: HorizontalPodAutoscaler
metadata:
name: springboot-app-hpa
spec:
scaleTargetRef:
apiVersion: apps/v1
kind: Deployment
name: springboot-app
minReplicas: 2
maxReplicas: 5
metrics:
- type: Resource
resource:
name: cpu
target:
type: Utilization
averageUtilization: 50
Apply HPA:
kubectl apply -f hpa.yaml
Step 9: CI/CD Pipeline with GitHub Actions (Optional)
Create .github/workflows/deploy.yml
:
name: Build and Deploy to AKS
on:
push:
branches: [ main ]
env:
ACR_NAME: springbootaksacr
RESOURCE_GROUP: springboot-aks-rg
CLUSTER_NAME: springboot-aks-cluster
APP_NAME: springboot-aks-demo
jobs:
build-and-deploy:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v2
- name: Set up JDK 17
uses: actions/setup-java@v2
with:
java-version: '17'
distribution: 'temurin'
- name: Build with Maven
run: mvn clean package -DskipTests
- name: Login to Azure
uses: azure/login@v1
with:
creds: ${{ secrets.AZURE_CREDENTIALS }}
- name: Build and push Docker image
run: |
az acr build --registry $ACR_NAME --image $APP_NAME:${{ github.sha }} .
- name: Deploy to AKS
run: |
az aks get-credentials --resource-group $RESOURCE_GROUP --name $CLUSTER_NAME
kubectl set image deployment/springboot-app springboot-app=$ACR_NAME.azurecr.io/$APP_NAME:${{ github.sha }} --record
Step 10: Monitoring and Logging
11.1 Enable Azure Monitor for Containers
az aks enable-addons -a monitoring -n springboot-aks-cluster -g springboot-aks-rg
11.2 View Logs
kubectl logs -f <pod-name>
11.3 Access Kubernetes Dashboard
az aks browse --resource-group springboot-aks-rg --name springboot-aks-cluster
Step 11: Clean Up Resources
When you're done, delete the resources to avoid ongoing charges:
az group delete --name springboot-aks-rg --yes --no-wait
Best Practices for Production
- Use Pod Disruption Budgets: Ensure high availability during maintenance
- Implement Network Policies: Control traffic between pods
- Configure Resource Quotas: Prevent resource exhaustion
- Use Azure Key Vault: For secrets management
- Implement Blue-Green Deployments: For zero-downtime updates
- Configure Backup: For your cluster configuration and persistent data
- Enable Cluster Autoscaler: To automatically adjust node count based on demand
This comprehensive guide covers the end-to-end process of deploying a Spring Boot application on AKS, from initial setup to production-grade configurations.