This comprehensive guide will walk you through building a production-ready RESTful API using Python, Flask, and Amazon DynamoDB, including Postman testing and unit testing.
1. Project Setup
Prerequisites
- Python 3.8+
- AWS account with DynamoDB access
- Postman (for API testing)
- Basic knowledge of REST principles
Initialize the Project
# Create project directory
mkdir flask-dynamodb-api
cd flask-dynamodb-api
# Create virtual environment
python -m venv venv
source venv/bin/activate # On Windows: venv\Scripts\activate
# Install required packages
pip install flask boto3 python-dotenv pytest pytest-cov
Project Structure
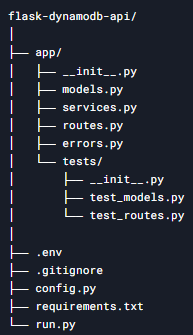
2. Finding AWS Credentials and Region Information
1. AWS Access Key ID and Secret Access Key
For IAM Users:
- Log in to the AWS Management Console (https://console.aws.amazon.com)
- Navigate to the IAM (Identity and Access Management) service
- In the left sidebar, click on "Users"
- Select your IAM user (or create one if you haven't)
- Click on the "Security credentials" tab
- Scroll down to the "Access keys" section
- Click "Create access key" if you don't have one
- In the popup, you'll see:
- Access key ID (starts with
AKIA...
) - Secret access key (only shown once - copy it immediately)
- Access key ID (starts with
⚠️ Important Security Notes:
- Never share your secret access key
- Store it securely (consider using AWS Secrets Manager)
- Rotate keys regularly (every 90 days is recommended)
- Never commit these keys to version control
Alternative for Root User (Not Recommended):
- Click on your account name in the top right
- Select "Security credentials"
- Scroll to "Access keys" section
- Create or view keys (but better to use IAM users)
2. AWS Region
The region is the geographical location where your AWS resources are hosted. Common regions include:
us-east-1
(N. Virginia)us-west-2
(Oregon)eu-west-1
(Ireland)ap-southeast-1
(Singapore)
How to check your region:
- Look at the URL in your AWS console:
console.aws.amazon.com/ec2/v2/home?region=us-east-1
showsus-east-1
- Or check the region selector in the top right of the console
3. Best Practices for Managing Credentials
Instead of hardcoding credentials in your application, consider these approaches:
Option A: AWS Credentials File
Create a file at ~/.aws/credentials
(Linux/Mac) or %UserProfile%\.aws\credentials
(Windows):
[default]
aws_access_key_id = YOUR_ACCESS_KEY
aws_secret_access_key = YOUR_SECRET_KEY
region = us-east-1
Then your Python code can simply use:
import boto3
client = boto3.client('dynamodb') # Automatically uses credentials from file
Option B: Environment Variables
Set them in your shell:
export AWS_ACCESS_KEY_ID="YOUR_ACCESS_KEY"
export AWS_SECRET_ACCESS_KEY="YOUR_SECRET_KEY"
export AWS_DEFAULT_REGION="us-east-1"
Option C: IAM Roles (Best for AWS deployments)
When running on EC2, Lambda, ECS, etc., assign an IAM role instead of using keys.
4. Verifying Your Credentials
Test your credentials work with this Python script:
import boto3
# Create a client
sts = boto3.client(
'sts',
aws_access_key_id='YOUR_ACCESS_KEY',
aws_secret_access_key='YOUR_SECRET_KEY',
region_name='us-east-1'
)
# Call AWS to verify
try:
response = sts.get_caller_identity()
print("Success! Your AWS account ID is:", response['Account'])
except Exception as e:
print("Error:", e)
5. Security Recommendations
- Never use root account credentials for applications
- Follow the principle of least privilege - give only the permissions needed
- Rotate credentials regularly (AWS recommends every 90 days)
- Use temporary credentials (AWS STS) when possible
- Set up MFA for extra security
- Monitor usage with AWS CloudTrail
6. Troubleshooting Common Issues
If you get errors like:
InvalidClientTokenId
: Your access key is incorrectSignatureDoesNotMatch
: Your secret key is incorrectUnrecognizedClientException
: Your credentials are disabledRequestExpired
: Your temporary credentials have expired
3. Configuration and Application Factory
Configuration (config.py)
import os
from dotenv import load_dotenv
load_dotenv()
class Config:
DEBUG = os.getenv('FLASK_DEBUG', 'False') == 'True'
AWS_ACCESS_KEY_ID = os.getenv('AWS_ACCESS_KEY_ID')
AWS_SECRET_ACCESS_KEY = os.getenv('AWS_SECRET_ACCESS_KEY')
AWS_REGION = os.getenv('AWS_REGION', 'us-east-1')
DYNAMODB_TABLE = os.getenv('DYNAMODB_TABLE', 'flask-api-items')
@staticmethod
def init_app(app):
pass
class DevelopmentConfig(Config):
DEBUG = True
class TestingConfig(Config):
TESTING = True
DYNAMODB_TABLE = 'test-flask-api-items'
config = {
'development': DevelopmentConfig,
'testing': TestingConfig,
'default': DevelopmentConfig
}
Application Factory (app/init.py)
from flask import Flask
from .routes import api_blueprint
from .errors import register_error_handlers
def create_app(config_name='default'):
app = Flask(__name__)
# Load configuration
from config import config
app.config.from_object(config[config_name])
# Initialize extensions
from .models import dynamodb
dynamodb.init_app(app)
# Register blueprints
app.register_blueprint(api_blueprint, url_prefix='/api/v1')
# Register error handlers
register_error_handlers(app)
return app
4. DynamoDB Integration
DynamoDB Model (app/models.py)
import boto3
from flask import current_app
from boto3.dynamodb.conditions import Key
class DynamoDB:
def __init__(self, app=None):
self.app = app
if app is not None:
self.init_app(app)
def init_app(self, app):
self.resource = boto3.resource(
'dynamodb',
aws_access_key_id=app.config['AWS_ACCESS_KEY_ID'],
aws_secret_access_key=app.config['AWS_SECRET_ACCESS_KEY'],
region_name=app.config['AWS_REGION']
)
self.table = self.resource.Table(app.config['DYNAMODB_TABLE'])
def get_item(self, item_id):
response = self.table.get_item(Key={'id': item_id})
return response.get('Item')
def create_item(self, item_data):
response = self.table.put_item(Item=item_data)
return response
def update_item(self, item_id, update_data):
update_expression = []
expression_attribute_values = {}
for key, value in update_data.items():
update_expression.append(f"{key} = :{key}")
expression_attribute_values[f":{key}"] = value
response = self.table.update_item(
Key={'id': item_id},
UpdateExpression='SET ' + ', '.join(update_expression),
ExpressionAttributeValues=expression_attribute_values,
ReturnValues='ALL_NEW'
)
return response.get('Attributes')
def delete_item(self, item_id):
response = self.table.delete_item(Key={'id': item_id})
return response
def scan_items(self):
response = self.table.scan()
return response.get('Items', [])
dynamodb = DynamoDB()
Service Layer (app/services.py)
from .models import dynamodb
from .errors import NotFoundError
class ItemService:
@staticmethod
def get_all_items():
return dynamodb.scan_items()
@staticmethod
def get_item(item_id):
item = dynamodb.get_item(item_id)
if not item:
raise NotFoundError(f"Item with id {item_id} not found")
return item
@staticmethod
def create_item(item_data):
if not item_data.get('id'):
raise ValueError("Item must have an 'id' field")
dynamodb.create_item(item_data)
return item_data
@staticmethod
def update_item(item_id, update_data):
item = ItemService.get_item(item_id)
updated_item = dynamodb.update_item(item_id, update_data)
return updated_item
@staticmethod
def delete_item(item_id):
ItemService.get_item(item_id) # Verify item exists
dynamodb.delete_item(item_id)
return {'message': 'Item deleted successfully'}
5. Implementing RESTful Endpoints
Routes (app/routes.py)
from flask import Blueprint, request, jsonify
from .services import ItemService
from .errors import NotFoundError, BadRequestError
api_blueprint = Blueprint('api', __name__)
@api_blueprint.route('/items', methods=['GET'])
def get_items():
items = ItemService.get_all_items()
return jsonify(items)
@api_blueprint.route('/items/<string:item_id>', methods=['GET'])
def get_item(item_id):
item = ItemService.get_item(item_id)
return jsonify(item)
@api_blueprint.route('/items', methods=['POST'])
def create_item():
data = request.get_json()
if not data:
raise BadRequestError("No input data provided")
item = ItemService.create_item(data)
return jsonify(item), 201
@api_blueprint.route('/items/<string:item_id>', methods=['PUT'])
def update_item(item_id):
data = request.get_json()
if not data:
raise BadRequestError("No input data provided")
updated_item = ItemService.update_item(item_id, data)
return jsonify(updated_item)
@api_blueprint.route('/items/<string:item_id>', methods=['DELETE'])
def delete_item(item_id):
response = ItemService.delete_item(item_id)
return jsonify(response), 200
Error Handling (app/errors.py)
from flask import jsonify
from werkzeug.exceptions import HTTPException
class APIError(HTTPException):
def __init__(self, message, status_code=400, payload=None):
super().__init__()
self.message = message
self.status_code = status_code
self.payload = payload
def to_dict(self):
rv = dict(self.payload or ())
rv['message'] = self.message
return rv
class NotFoundError(APIError):
def __init__(self, message):
super().__init__(message, 404)
class BadRequestError(APIError):
def __init__(self, message):
super().__init__(message, 400)
def register_error_handlers(app):
@app.errorhandler(APIError)
def handle_api_error(error):
response = jsonify(error.to_dict())
response.status_code = error.status_code
return response
@app.errorhandler(404)
def handle_not_found(error):
response = jsonify({'message': 'Resource not found'})
response.status_code = 404
return response
@app.errorhandler(500)
def handle_server_error(error):
response = jsonify({'message': 'Internal server error'})
response.status_code = 500
return response
6. Postman Testing
Setting Up Postman
- Create a new collection called "Flask DynamoDB API"
- Set the base URL as
http://localhost:5000/api/v1
Sample Requests
Create Item (POST /items)
{
"id": "item1",
"name": "Sample Item",
"description": "This is a sample item",
"price": 9.99
}
Get All Items (GET /items)
Get Single Item (GET /items/item1)
Update Item (PUT /items/item1)
{
"name": "Updated Item",
"price": 12.99
}
Delete Item (DELETE /items/item1)
7. Unit Testing
Test Setup (app/tests/init.py)
import pytest
from app import create_app
from app.models import dynamodb
@pytest.fixture
def app():
app = create_app('testing')
with app.app_context():
yield app
@pytest.fixture
def client(app):
return app.test_client()
@pytest.fixture
def dynamodb_table(app):
# Create test table
dynamodb.resource.create_table(
TableName=app.config['DYNAMODB_TABLE'],
KeySchema=[{'AttributeName': 'id', 'KeyType': 'HASH'}],
AttributeDefinitions=[{'AttributeName': 'id', 'AttributeType': 'S'}],
ProvisionedThroughput={'ReadCapacityUnits': 1, 'WriteCapacityUnits': 1}
)
yield
# Clean up
dynamodb.resource.Table(app.config['DYNAMODB_TABLE']).delete()
Model Tests (app/tests/test_models.py)
def test_create_and_get_item(dynamodb_table):
test_item = {'id': 'test1', 'name': 'Test Item'}
dynamodb.create_item(test_item)
item = dynamodb.get_item('test1')
assert item == test_item
def test_update_item(dynamodb_table):
test_item = {'id': 'test1', 'name': 'Test Item'}
dynamodb.create_item(test_item)
updated = dynamodb.update_item('test1', {'name': 'Updated Name'})
assert updated['name'] == 'Updated Name'
def test_delete_item(dynamodb_table):
test_item = {'id': 'test1', 'name': 'Test Item'}
dynamodb.create_item(test_item)
dynamodb.delete_item('test1')
assert dynamodb.get_item('test1') is None
Route Tests (app/tests/test_routes.py)
import json
def test_create_item(client, dynamodb_table):
response = client.post('/items', json={
'id': 'test1',
'name': 'Test Item'
})
assert response.status_code == 201
assert b'test1' in response.data
def test_get_item(client, dynamodb_table):
# First create an item
client.post('/items', json={'id': 'test1', 'name': 'Test Item'})
response = client.get('/items/test1')
assert response.status_code == 200
data = json.loads(response.data)
assert data['name'] == 'Test Item'
def test_get_nonexistent_item(client, dynamodb_table):
response = client.get('/items/nonexistent')
assert response.status_code == 404
def test_update_item(client, dynamodb_table):
# Create item first
client.post('/items', json={'id': 'test1', 'name': 'Test Item'})
response = client.put('/items/test1', json={'name': 'Updated Name'})
assert response.status_code == 200
data = json.loads(response.data)
assert data['name'] == 'Updated Name'
def test_delete_item(client, dynamodb_table):
# Create item first
client.post('/items', json={'id': 'test1', 'name': 'Test Item'})
response = client.delete('/items/test1')
assert response.status_code == 200
# Verify it's gone
response = client.get('/items/test1')
assert response.status_code == 404
Running Tests
# Run tests with coverage
pytest --cov=app tests/
# Generate HTML coverage report
pytest --cov=app --cov-report=html tests/
7. Deployment Considerations
Environment Variables (.env)
FLASK_DEBUG=True
AWS_ACCESS_KEY_ID=your_access_key
AWS_SECRET_ACCESS_KEY=your_secret_key
AWS_REGION=us-east-1
DYNAMODB_TABLE=flask-api-items
Production Considerations
- DynamoDB Provisioning: Adjust read/write capacity based on expected load
- Error Handling: Implement more sophisticated error logging
- Authentication: Add JWT or API key authentication
- Rate Limiting: Implement to prevent abuse
- CORS: Configure properly if your API will be accessed from web apps
- WSGI Server: Use Gunicorn or uWSGI for production
Example Deployment with Gunicorn
pip install gunicorn
gunicorn -w 4 -b :5000 run:app
Example run.py
from app import create_app
app = create_app()
if __name__ == '__main__':
app.run()
This comprehensive guide provides everything you need to build, test, and deploy a production-ready RESTful API with Flask and DynamoDB. The architecture follows best practices with clear separation of concerns, proper error handling, and thorough testing.