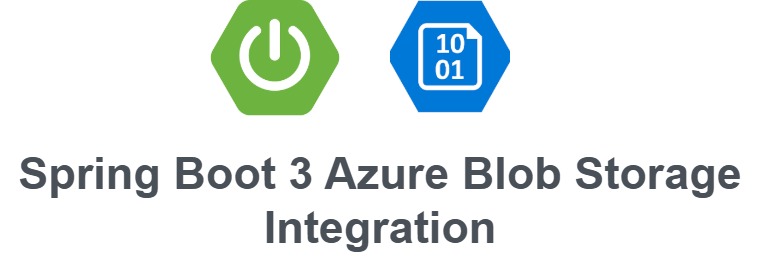
Azure Blob Storage
Overview:
Azure Blob Storage is a cloud-based object storage service from Microsoft Azure, designed for storing massive amounts of unstructured data such as images, videos, documents, and backups.
Key Features:
- Scalable & Durable – Handles petabytes of data with high availability.
- Secure – Supports encryption, role-based access control (RBAC), and private networks.
- Cost-Effective – Offers multiple storage tiers (Hot, Cool, and Archive) for cost optimization.
- Easy Integration – Works seamlessly with Azure services, SDKs, and REST APIs.
Common Use Cases:
- Storing and serving static content (e.g., images, videos).
- Data backup and disaster recovery.
- Logging and analytics.
- Cloud-based file sharing.
Spring Cloud Azure Starter Storage (spring-cloud-azure-starter-storage
)
Overview:
The spring-cloud-azure-starter-storage
dependency is a part of the Spring Cloud Azure project, designed to seamlessly integrate Azure Blob Storage with Spring Boot applications. It simplifies working with Azure Storage by providing auto-configuration, connection handling, and easy-to-use APIs.
Key Features:
- Simplified Configuration – Automatically connects to Azure Blob Storage using properties from
application.properties
. - Spring Boot Integration – Works with Spring’s dependency injection and environment properties.
- Blob Operations – Supports file upload, download, delete, and listing blobs with minimal boilerplate code.
- Security & Authentication – Supports Managed Identity, SAS tokens, and Connection Strings for secure access.
Example Use Case:
Using this starter, you can easily create REST APIs in Spring Boot to store, retrieve, and manage files in Azure Blob Storage without dealing with low-level SDK complexities.
Here's a complete example for file upload, download, and delete operations using Azure Blob Storage with Spring Boot.
Complete POM.xml
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 https://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>3.4.4</version>
<relativePath/> <!-- lookup parent from repository -->
</parent>
<groupId>com.example</groupId>
<artifactId>azure-blob-demo</artifactId>
<version>0.0.1-SNAPSHOT</version>
<name>azure-blob-demo</name>
<description>Demo project for Azure Blob Storage with Spring Boot</description>
<properties>
<java.version>17</java.version>
<spring-cloud-azure.version>5.21.0</spring-cloud-azure.version>
</properties>
<dependencies>
<!-- Spring Web -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<!-- Azure Storage -->
<dependency>
<groupId>com.azure.spring</groupId>
<artifactId>spring-cloud-azure-starter-storage</artifactId>
</dependency>
<!-- Lombok -->
<dependency>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
<optional>true</optional>
</dependency>
<!-- For testing -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
</dependencies>
<dependencyManagement>
<dependencies>
<dependency>
<groupId>com.azure.spring</groupId>
<artifactId>spring-cloud-azure-dependencies</artifactId>
<version>${spring-cloud-azure.version}</version>
<type>pom</type>
<scope>import</scope>
</dependency>
</dependencies>
</dependencyManagement>
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
</plugin>
</plugins>
</build>
</project>
Application Configuration
1. Find Your Storage Account Name
- Log in to the Azure Portal
- Navigate to "Storage accounts"
- Select your storage account
- The account name is displayed at the top of the overview page
2. Find Your Storage Account Key
- In your storage account, go to "Security + networking" > "Access keys"
- You'll see two keys (key1 and key2) - you can use either one
- Click the "Show" button to reveal the key
- Copy either "Key1" or "Key2" value
3. Create or Find Your Container Name
- In your storage account, go to "Data storage" > "Containers"
- Click "+ Container" to create a new one if needed
- Enter a name (must be lowercase, numbers, and hyphens only)
- Set the public access level (typically "Private" for most applications)
- Click "Create"
4. Construct the Endpoint URL
https://<your-storage-account-name>.blob.core.windows.net
Just replace <your-storage-account-name>
with your actual storage account name.
Complete Configuration Example
Based on the values you find, your application.properties
should look like:
# Azure Storage Configuration
spring.cloud.azure.storage.blob.account-name=mystorageaccount123
spring.cloud.azure.storage.blob.account-key=AbCdEfGhIjKlMnOpQrStUvWxYz0123456789+ABCDEFGHIJKLMNOPQRSTUVWXYZ1234567890+ABCDEFG==
spring.cloud.azure.storage.blob.container-name=my-container
spring.cloud.azure.storage.blob.endpoint=https://mystorageaccount123.blob.core.windows.net
Important Security Notes:
- Never commit your account key to version control - Use environment variables or Azure Key Vault in production:
spring.cloud.azure.storage.blob.account-key=${AZURE_STORAGE_KEY}
- For production, consider using Managed Identity instead of account keys:
spring.cloud.azure.storage.blob.managed-identity.client-id=<your-managed-identity-client-id>
- Best practice: Create a separate storage account for development/testing different from production.
Alternative: Find Connection String
If you prefer using a connection string:
- Go to "Access keys" in your storage account
- Copy the "Connection string"
- Use this in your properties:
spring.cloud.azure.storage.blob.connection-string=DefaultEndpointsProtocol=https;AccountName=mystorageaccount123;AccountKey=AbCdEf...==;EndpointSuffix=core.windows.net
Azure Blob Storage Service
Create a service class to handle blob operations:
import com.azure.storage.blob.BlobClient;
import com.azure.storage.blob.BlobContainerClient;
import com.azure.storage.blob.BlobServiceClient;
import com.azure.storage.blob.models.BlobItem;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import org.springframework.web.multipart.MultipartFile;
import java.io.ByteArrayOutputStream;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
@Service
public class AzureBlobStorageService {
@Autowired
private BlobServiceClient blobServiceClient;
@Autowired
private BlobContainerClient blobContainerClient;
public String uploadFile(MultipartFile file) throws IOException {
// Create blob client for the file
BlobClient blobClient = blobContainerClient.getBlobClient(file.getOriginalFilename());
// Upload the file
blobClient.upload(file.getInputStream(), file.getSize(), true);
return "File uploaded successfully: " + file.getOriginalFilename();
}
public byte[] downloadFile(String fileName) {
BlobClient blobClient = blobContainerClient.getBlobClient(fileName);
ByteArrayOutputStream outputStream = new ByteArrayOutputStream();
blobClient.download(outputStream);
return outputStream.toByteArray();
}
public String deleteFile(String fileName) {
BlobClient blobClient = blobContainerClient.getBlobClient(fileName);
blobClient.delete();
return "File deleted successfully: " + fileName;
}
public List<String> listFiles() {
List<String> fileNames = new ArrayList<>();
for (BlobItem blobItem : blobContainerClient.listBlobs()) {
fileNames.add(blobItem.getName());
}
return fileNames;
}
}
REST Controller
Create a controller to expose the endpoints:
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.core.io.ByteArrayResource;
import org.springframework.http.HttpHeaders;
import org.springframework.http.MediaType;
import org.springframework.http.ResponseEntity;
import org.springframework.web.bind.annotation.*;
import org.springframework.web.multipart.MultipartFile;
import java.io.IOException;
import java.util.List;
@RestController
@RequestMapping("/api/files")
public class FileController {
@Autowired
private AzureBlobStorageService azureBlobStorageService;
@PostMapping("/upload")
public String uploadFile(@RequestParam("file") MultipartFile file) throws IOException {
return azureBlobStorageService.uploadFile(file);
}
@GetMapping("/download/{fileName}")
public ResponseEntity<ByteArrayResource> downloadFile(@PathVariable String fileName) {
byte[] data = azureBlobStorageService.downloadFile(fileName);
ByteArrayResource resource = new ByteArrayResource(data);
return ResponseEntity.ok()
.header(HttpHeaders.CONTENT_DISPOSITION, "attachment;filename=" + fileName)
.contentType(MediaType.APPLICATION_OCTET_STREAM)
.contentLength(data.length)
.body(resource);
}
@DeleteMapping("/delete/{fileName}")
public String deleteFile(@PathVariable String fileName) {
return azureBlobStorageService.deleteFile(fileName);
}
@GetMapping("/list")
public List<String> listFiles() {
return azureBlobStorageService.listFiles();
}
}
Main Application Class
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class AzureBlobDemoApplication {
public static void main(String[] args) {
SpringApplication.run(AzureBlobDemoApplication.class, args);
}
}
How to Use
1. Upload a file:
POST /api/files/upload
Content-Type: multipart/form-data
Body: file=[your-file]
2. Download a file:
GET /api/files/download/{fileName}
3. Delete a file:
DELETE /api/files/delete/{fileName}
4. List all files:
GET /api/files/list
Notes
- Make sure your Azure Storage account allows the operations you're trying to perform
- For production, consider using Azure Managed Identity instead of account keys
- Add proper error handling and logging in production code
- Consider adding file size limits and content type validation
☁️ Spring Boot 3 Azure Blob Storage Integration
Learn how to integrate Azure Blob Storage with Spring Boot 3 for file upload, download, listing, and deletion.
📂 Clone on GitHub